Introduction
As technology evolves, developers often face the challenge of integrating legacy systems with modern platforms. One of the most common scenarios is bridging the gap between applications built using the .NET Framework and those leveraging the latest advancements in new .NET Core and .NET solutions.
Learn more about .NET and .NET Core at Microsoft .NET Docs.
Learn more about .NET Framework at Microsoft .NET Framework Docs
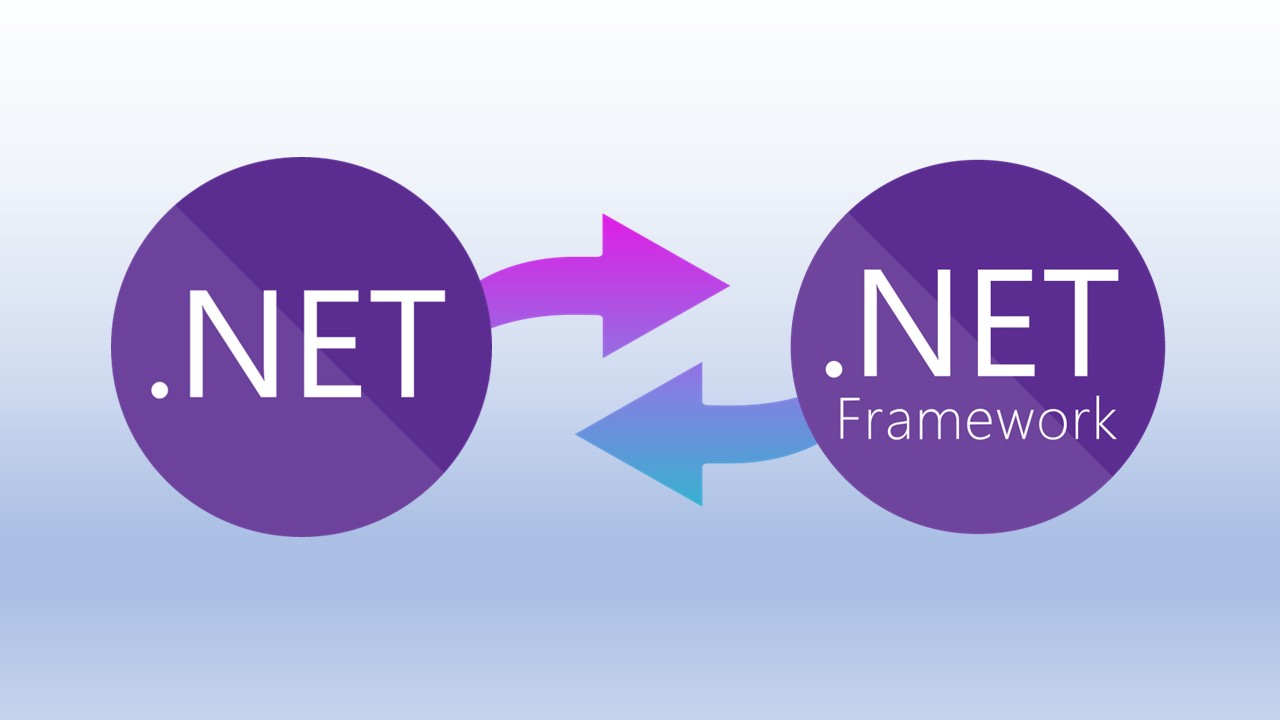
The .NET Framework, with its rich history and extensive library support, continues to power countless enterprise solutions. However, as organizations embrace the performance, scalability, and cross-platform benefits of .NET Core and its successors, the need for seamless interoperability becomes critical.
This is where Javonet comes into play. Javonet provides a powerful solution to enable direct communication between those two technologies, eliminating the complexities and inefficiencies of traditional integration approaches. Whether you’re looking to reuse legacy libraries, modernize existing systems, or extend functionality across platforms, Javonet simplifies the process, saving time and effort while maintaining the integrity of your codebase.
In this article, we’ll explore how Javonet enables smooth interaction between .NET Framework and .NET, and why it’s a game-changer for developers seeking to future-proof their applications without rewriting legacy code from scratch. We will show how you can call .NET Framework code from .NET 8 and the other way around (call .NET 8 from .NET Framework)
Activating Javonet
If you haven’t installed Javonet yet, here are the exact instructions for .NET Javonet Documentation – .NET Quickstart Guide.
Calling .NET Framework from .NET 8 console application
.NET Framework code
Let’s create class library for .NET Framework with 2 classes:
using System;
namespace ClassLibrary
{
public class TestClass
{
public void TestMethod()
{
Console.WriteLine("Hello from .NET Framework class!");
}
}
}
using System;
namespace ClassLibrary
{
public static class TestStaticClass
{
public static void TestMethod()
{
Console.WriteLine("Hello from .NET Framework static class!");
}
}
}
We need to build the code and locate the gener/bin/Release/
/bin/Debug/
`ClassLibrary.dll.
.NET 8 code
Now, let’s create a .NET 8.0 console app that will call methods created above.
Due to some technical limitations of Javonet we have to use classic Main()
structure of Program.cs
On the first line, we can add Console.WriteLine()
to display the source of the data, activate Javonet, and initialize the .NET Framework runtime:
Console.WriteLine("Hello, World from .NET code!");
Javonet.Activate("your-license-key");
RuntimeContext calledRuntime = Javonet.InMemory().Clr();
In the next step, we need to provide the path to our .NET Framework DLL file.
calledRuntime.LoadLibrary("Your/DLL/File/Path");
Once the runtime is created, we can begin calling methods. To invoke an instance method, we first need to create an instance of the class…
InvocationContext calledRuntimeType = calledRuntime.GetType("ClassLibrary.TestClass").Execute();
… and call a method:
InvocationContext instance = calledRuntimeType.CreateInstance().Execute();
instance.InvokeInstanceMethod("TestMethod").Execute();
For static methods we can use:
InvocationContext calledStaticRuntime = calledRuntime.GetType("ClassLibrary.TestStaticClass").Execute();
calledStaticRuntime.InvokeStaticMethod("TestMethod").Execute();
Our final code may look like below:
namespace JavonetTest
{
using Javonet.Netcore.Sdk;
internal class Program
{
private static void Main(string[] args)
{
Console.WriteLine("Hello from .NET code!");
Javonet.Activate("your-license-key");
RuntimeContext calledRuntime = Javonet.InMemory().Clr();
string libraryPath = "Your/DLL/File/Path";
string className = "ClassLibrary.TestClass";
string staticClassName = "ClassLibrary.TestStaticClass";
calledRuntime.LoadLibrary(libraryPath);
InvocationContext calledRuntimeType = calledRuntime.GetType(className).Execute();
InvocationContext instance = calledRuntimeType.CreateInstance().Execute();
instance.InvokeInstanceMethod("TestMethod").Execute();
InvocationContext calledStaticRuntime = calledRuntime.GetType(staticClassName).Execute();
calledStaticRuntime.InvokeStaticMethod("TestMethod").Execute();
}
}
}
After executing this code, the console output should look similar to the example below:
Hello, World from .NET code!
javonet.lic exists. Verifying... License valid.
Hello from .NET Framework class!
Hello from .NET Framework static class!
Calling .NET 8 from .NET Framework application
The process here will be quite similar. First, we need to create a .NET 8 class library
.
.NET 8 code
Let’s create 2 classes:
namespace NetCoreClassLibrary
{
public class TestClass
{
public string TestMethod(string name)
{
return $"Hello from .NET 8.0 class, {name}!";
}
}
}
and:
namespace NetCoreClassLibrary
{
public static class TestStaticClass
{
public static string TestMethod(string name)
{
return $"Hello from .NET 8.0 static class, {name}!";
}
}
}
.NET Framework code
Our code here has an almost identical structure. However, this time, we need to add an argument to the method invocation. The code might look like this:
using System;
namespace FrameworkConsoleApp
{
using Javonet.Clr.Sdk;
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World from .NET Framework code!");
Javonet.Activate("your-license-key");
RuntimeContext calledRuntime = Javonet.InMemory().Netcore();
string libraryPath = "Your/DLL/File/Path";
string className = "NetCoreClassLibrary.TestClass";
string staticClassName = "NetCoreClassLibrary.TestStaticClass";
calledRuntime.LoadLibrary(libraryPath);
InvocationContext calledRuntimeType = calledRuntime.GetType(className).Execute();
InvocationContext instance = calledRuntimeType.CreateInstance().Execute();
InvocationContext response = instance.InvokeInstanceMethod("TestMethod", "User").Execute();
Console.WriteLine(response.GetValue());
InvocationContext calledRuntimeTypeForStatic = calledRuntime.GetType(staticClassName).Execute();
InvocationContext staticResponse = calledRuntimeTypeForStatic.InvokeStaticMethod("TestMethod", "User").Execute();
Console.WriteLine(staticResponse.GetValue());
}
}
}
As you can see, we passed an argument to the methods as the second parameter of InvokeInstanceMethod()
and InvokeStaticMethod()
.
Conclusion
Bridging the gap between .NET Framework and .NET Core, including .NET 8, doesn’t have to be a challenge. With Javonet, you can seamlessly integrate legacy code with modern applications, saving time and resources while future-proofing your projects.
Ready to simplify your development process? Try Javonet today and unlock the full potential of your .NET ecosystem!
Introduction
As technology evolves, developers often face the challenge of integrating legacy systems with modern platforms. One of the most common scenarios is bridging the gap between applications built using the .NET Framework and those leveraging the latest advancements in new .NET Core and .NET solutions.
Learn more about .NET and .NET Core at Microsoft .NET Docs.
Learn more about .NET Framework at Microsoft .NET Framework Docs
The .NET Framework, with its rich history and extensive library support, continues to power countless enterprise solutions. However, as organizations embrace the performance, scalability, and cross-platform benefits of .NET Core and its successors, the need for seamless interoperability becomes critical.
This is where Javonet comes into play. Javonet provides a powerful solution to enable direct communication between those two technologies, eliminating the complexities and inefficiencies of traditional integration approaches. Whether you’re looking to reuse legacy libraries, modernize existing systems, or extend functionality across platforms, Javonet simplifies the process, saving time and effort while maintaining the integrity of your codebase.
In this article, we’ll explore how Javonet enables smooth interaction between .NET Framework and .NET, and why it’s a game-changer for developers seeking to future-proof their applications without rewriting legacy code from scratch. We will show how you can call .NET Framework code from .NET 8 and the other way around (call .NET 8 from .NET Framework)
Activating Javonet
If you haven’t installed Javonet yet, here are the exact instructions for .NET Javonet Documentation – .NET Quickstart Guide.
Calling .NET Framework from .NET 8 console application
.NET Framework code
Let’s create class library for .NET Framework with 2 classes:
We need to build the code and locate the generated DLL fileIt should be in
/bin/Release/
or/bin/Debug/
directory. In this case, the file will be named `ClassLibrary.dll..NET 8 code
Now, let’s create a .NET 8.0 console app that will call methods created above.
Due to some technical limitations of Javonet we have to use classic
Main()
structure ofProgram.cs
On the first line, we can add
Console.WriteLine()
to display the source of the data, activate Javonet, and initialize the .NET Framework runtime:In the next step, we need to provide the path to our .NET Framework DLL file.
Once the runtime is created, we can begin calling methods. To invoke an instance method, we first need to create an instance of the class…
… and call a method:
For static methods we can use:
Our final code may look like below:
After executing this code, the console output should look similar to the example below:
Calling .NET 8 from .NET Framework application
The process here will be quite similar. First, we need to create a .NET 8
class library
..NET 8 code
Let’s create 2 classes:
and:
.NET Framework code
Our code here has an almost identical structure. However, this time, we need to add an argument to the method invocation. The code might look like this:
As you can see, we passed an argument to the methods as the second parameter of
InvokeInstanceMethod()
andInvokeStaticMethod()
.Conclusion
Bridging the gap between .NET Framework and .NET Core, including .NET 8, doesn’t have to be a challenge. With Javonet, you can seamlessly integrate legacy code with modern applications, saving time and resources while future-proofing your projects.
Ready to simplify your development process? Try Javonet today and unlock the full potential of your .NET ecosystem!